Proximity sensing is very important in a number of applications including security systems, navigation systems, industrial automation and others. I have already looked at a number of sensors that can be used for proximity detection including PIR sensors and Ultrasonic sensors.
In this tutorial, we will explore the RCWL-0516 Microwave radar sensor, a low-cost device that uses Doppler radar for proximity detection. It can be used on its own or with a microcontroller like Arduino.
The Doppler Effect
The Doppler Effect explains the apparent change in the frequency of a wave caused by relative motion between the source of the wave and an observer.
The commonest example to demonstrate the Doppler Effect is the sound of an ambulance siren. When the ambulance is moving towards a stationary observer, the frequency of the sound waves increases and the sound will be higher in pitch.
Once the ambulance passes and is moving away from the observer, the frequency of the sound waves decreases and the sound is lower in pitch.
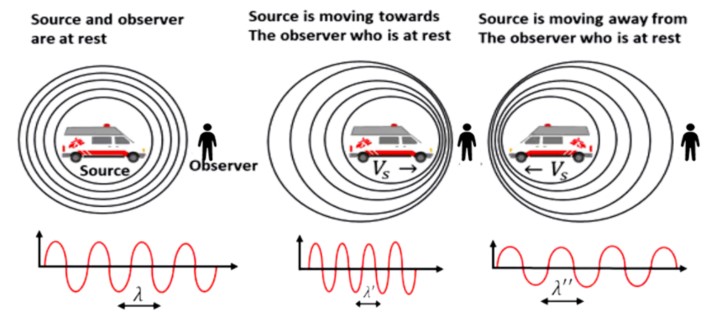
This phenomenon is used in a number of applications including astronomy, satellite communications, navigation in ships and aircraft and of course Doppler radar is used in RCWL-0516 sensor.
How does the RCWL-0516 Microwave Radar sensor work?
The RCWL-0516 sensor is an active sensor with a built-in oscillator that generates a microwave signal at a frequency of 3.18 GHz. The sensor then sends out this signal in a 360-degree pattern and measures the reflected signals.
When an object moves within the sensor’s range, the reflected waves are picked up by the sensor’s receiver. The receiver then measures the frequency of the reflected waves and compares it to the frequency of the original signal. If the frequency of the reflected waves has changed, the sensor knows that an object is in its range of detection.
Due to Doppler Effect, the frequency of the reflected microwave signal is different from the transmitted signal when an object is moving towards or away from the sensor
The RCWL-0516 sensor has a single output pin that goes HIGH when it detects movement. It outputs LOW when no motion is detected.
RCWL-0516 Microwave Radar Proximity Sensor Pinout
This sensor is built around a Doppler radar controller IC – RCWL-9196 which is very similar to the BISS0001 IC that is used in PIR sensors. This IC supports repeat triggers and has a 360-degree detection area without blind spots.
The RCWL-0516 sensor also has a microwave antenna integrated on the PCB connected to a MMBR941M RF power amplifier for boosting the low-power RF signal from the antenna.
The pinouts for the sensor are as shown below.

- 3V3: this is a 3.3V output, not a power supply input. The device has an integrated 3.3 volt voltage regulator which can provide up to 100 mA of current for powering external logic circuitry.
- VIN: the power input pin. The sensor can be powered by a voltage range of 4-28V.
- GND: ground pin.
- OUT: The output pin. The output pin goes HIGH when the sensor detects movement and remains LOW when it doesn’t.
- CDS: This pin is used to connect a light-dependent resistor (LDR). The LDR can be used to disable the sensor in bright light conditions.
CDS stands for Cadmium Sulphide, which is the photoactive component in LDRs. Because of this, LDRs are sometimes called CDS photo resistors.
You can connect the LDR to the sensor by either using the two CDS pads on the top of the module or by connecting one end of the LDR to the CDS pin at the terminal end, and the other end to the ground.
Adjusting the sensor’s default settings.
There are three solder jumpers on the back of this sensor that are used to adjust the pulse length, range of detection and light sensitivity
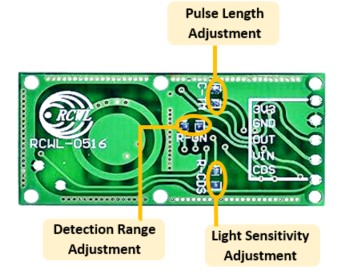
- C-TM: By placing a suitable SMD capacitor, you can adjust the output pulse length and therefore regulate the repeat trigger time. The higher the capacitor value the longer the pulse length. The default trigger time is 2s and if you put a 0.2µF capacitor, the time will be increased to 50s.
- R-GN: You can adjust the sensor’s detection range by placing a suitable resistor between these jumpers. The default detection range is 7m. Adding a 1M resistor reduces it to 5m while a 270kΩ resistor reduces the range to 1.5m.
- R-CDS: Without a resistor on this jumper, the lowest resistance of the LDR where the output is enabled is approximately 269kΩ. Adding resistance here decreases the LDR resistance of the enable/disable threshold. If the LDR resistance at the desired light level threshold is greater than 269kΩ then you could add an external resistor in series with the LDR.
Using RCWL-0516 sensor without Arduino
This sensor can be used without connecting it to any microcontroller, for example in the setup below I have connected an LED to the output via a 220Ω current limiting resistor and supplied the sensor with 5V.
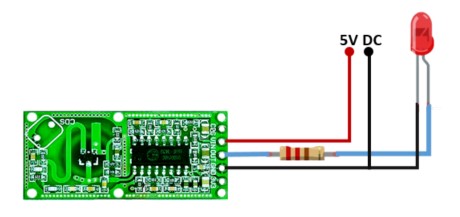
When you make a movement near the sensor, the LED will turn on for about two seconds and then turn off indicating that the sensor has detected the presence of an object.
I also tested the sensitivity of this sensor from different angles and when placed inside wooden and plastic enclosures and it worked just fine.
Adding a Light Dependent Resistor to RCWL-0516 sensor.
An LDR is a resistor whose resistance changes when exposed to light. LDRs are not polarity-sensitive so you can connect it to the sensor any way you want. You can either use the pads at the top of the RCWL-0516 or use the CDS pin on the bottom and connect your LDR between it and the ground connection.
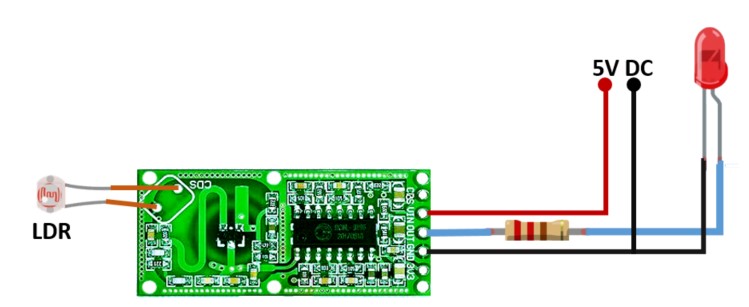
You will now observe that the sensor will not detect any objects near it when the LDR is exposed to light. But when the light is turned off, the sensor works normally. This has a very practical application in detection of intruders at night.
Using RCWL-0516 microwave radar sensor with Arduino
This sensor achieves most of its basic functionality without the need for a microcontroller for example triggering of lights or alarms in simple security systems using proximity detection.
However in some other cases, we may need to use the RCWL-0516 sensor in more complex setups for example if you need to receive security alerts from this sensor on your phone via a web page or sms, then you need to use microcontrollers to achieve such communication.
I will give a basic application of the RCWL-0516 sensor with Arduino as a latching device. When using this sensor without Arduino, whenever the proximity detector is triggered, the LED turns on and goes off after about two seconds. However to make this more practical for security applications, the LED should remain on until it is intentionally removed. This is where the idea of latching comes in.
The setup will be as shown below
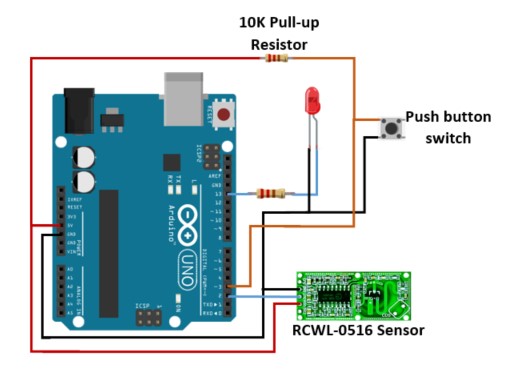
The output pin of RCWL-0516 sensor will be connected to a digital pin of Arduino. An LED is connected to digital pin 13 with a 220Ω dropping resistor and I have also included a tactile switch button at pin 3 with a 10k pull-up resistor.
The code sketch for the working of this setup is;
int Sensor = 2; // RCWL-0516 Input Pin
int Pbut = 3; // Push button Input Pin
int LED = 13; // LED Output Pin
int sensorval = 0; // RCWL-0516 Sensor Value
int pbval = 0; // Push button value
void setup() {
pinMode (Sensor, INPUT); // RCWL-0516 as input
pinMode (Pbut, INPUT); // Push button as input
pinMode (LED, OUTPUT); // LED as OUTPUT
digitalWrite(LED, LOW); // Turn LED Off
}
void loop(){
sensorval = digitalRead(Sensor); // Read Sensor value
pbval = digitalRead(Pbut); // Read Push button value
if (sensorval == HIGH) {
digitalWrite(LED, HIGH); // Turn LED On
}
if (pbval == LOW) {
digitalWrite(LED, LOW); // Turn LED Off
}
}
We begin by defining pins where the RCWL-0516 sensor, the LED and push-button are connected and also define variables to hold the values of the sensor and push button.
In the setup we declare the sensor and push-button connections as inputs and the LED connection as an output. We use the digitalWrite() function to turn off the LED.
In the loop, we first read the values for the RCWL-0516 sensor and push-button using digitalRead() function. If statements are used to set the state of the sensor, LED and push button. When the sensor is triggered it will read HIGH and the LED will turn on. When the push-button is pressed it will read LOW and the LED will turn off.
You can also connect the LDR so that it only works in the dark and instead of an LED you can use other devices like motors and relays for controlling several appliances whenever the RCWL-0516 sensor detects movement.