The Arduino CNC Shield V3 provides an inexpensive solution for controlling stepper motors in various DIY CNC machines, including CNC milling machines, laser engraving or cutting machines, drawing machines, 3D printers, and other projects requiring precise stepper motor control.
This shield fits onto the Arduino UN board, is compatible with Grbl, an Open source G-Code interpreter and uses various compatible stepper motor drivers including A4988 or the higher current DRV8825 and TMC driver modules.
Before using this CNC shield, you should know how to use the stepper motor drivers you intend to use. I have other detailed tutorials on the working of these drivers which you can first go through for reference;
Arduino CNC Shield Hardware Overview
You fit the Shield onto the Arduino UNO microcontroller and connect the motors directly to the output pins of the drivers. You choose the drivers based on the power of the motors and the application.
The main parts of the CNC shield are as shown below.
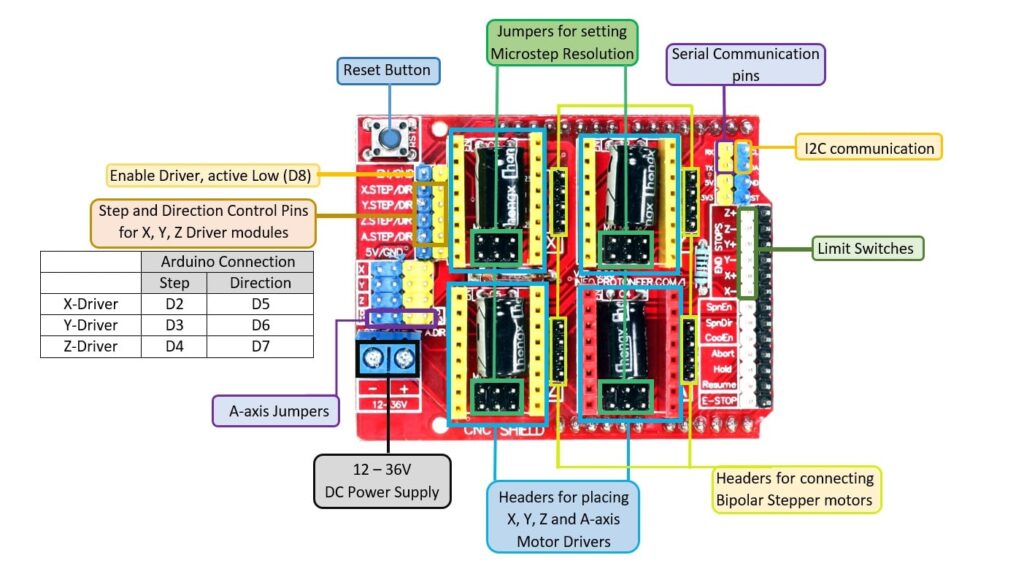
This shield runs on 12-36V DC power supply therefore you have to consider the operating voltage when powering the shield. For now, only DRV8825 drivers can handle up to 36V.
The shield includes a fourth A-axis driver, which can be configured to mirror any of the X, Y, or Z axes. It can also operate independently using pins D12 for A-STEP and D13 for A-DIR. You need to connect the A-axis jumpers labelled in the diagram above to be able to use the A-axis driver.
You can also check out a more detailed pinout of the CNC shield from the manufacturer’s website to further see how each of the pins on this shield relate to their corresponding Arduino pin connections.
Connect the CNC Shield, Arduino, motor drivers, and stepper motors as shown below.
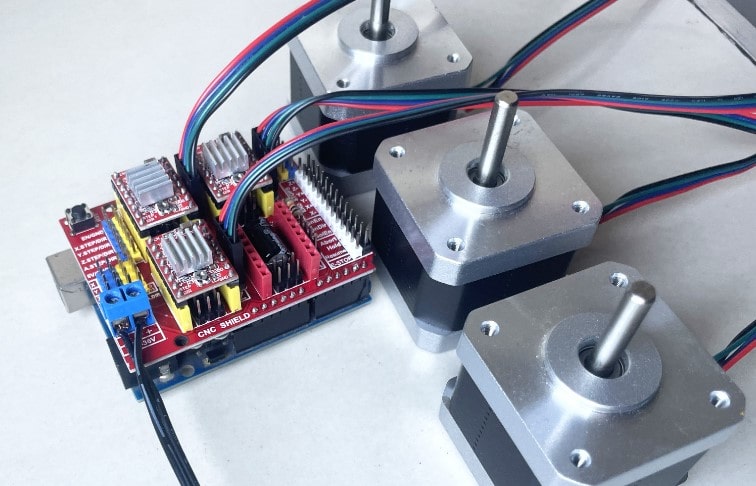
Check out for these before powering your CNC Shield.
Before proceeding to how to use this CNC shield, there are some things you should put into consideration other wise your CNC Shield may not work;
NEVER connect or disconnect any driver or stepper motor to the CNC Shield while power is on.
When installing the driver make sure to correctly orientate the driver so the enable pin (EN) matches the EN pin on the CNC Shield.
ALWAYS connect a stepper motor to the CNC Shield when testing or using the CNC Shield and driver. This is very important because the motor drivers are designed to ramp up current until they reach the level needed to run. Without a stepper motor connected there will be nothing to consume the current and you can end up damaging the stepper driver if it over-heats in the process
Be careful when fixing a heatsink to the drivers. You should place the heatsink in the middle, ensuring it doesn’t touch any of the driver pins, as this could cause a short that would damage the driver.
Make sure none of the motor drivers are faulty and that you have correctly set the current limit. If any of the drivers is faulty, the whole setup will not work.
Testing the CNC Shield
First, properly place the motor drivers on the CNC Shield. Before using the GRBL firmware, test the setup using the code sketch below to check if everything is working properly.
// defines pins numbers
const int stepX = 2;
const int dirX = 5;
const int stepY = 3;
const int dirY = 6;
const int stepZ = 4;
const int dirZ = 7;
const int enPin = 8;
void setup() {
// Sets the two pins as Outputs
pinMode(stepX,OUTPUT);
pinMode(dirX,OUTPUT);
pinMode(stepY,OUTPUT);
pinMode(dirY,OUTPUT);
pinMode(stepZ,OUTPUT);
pinMode(dirZ,OUTPUT);
pinMode(enPin,OUTPUT);
digitalWrite(enPin,LOW);
digitalWrite(dirX,HIGH);
digitalWrite(dirY,LOW);
digitalWrite(dirZ,HIGH);
}
void loop() {
// Enables the motor to move in a particular direction
// Makes 200 pulses for making one full cycle rotation
for(int x = 0; x < 800; x++) {
digitalWrite(stepX,HIGH);
delayMicroseconds(1000);
digitalWrite(stepX,LOW);
delayMicroseconds(1000);
}
delay(1000); // One second delay
for(int x = 0; x < 800; x++) {
digitalWrite(stepY,HIGH);
delayMicroseconds(1000);
digitalWrite(stepY,LOW);
delayMicroseconds(1000);
}
delay(1000); // One second delay
for(int x = 0; x < 800; x++) {
digitalWrite(stepZ,HIGH);
delayMicroseconds(1000);
digitalWrite(stepZ,LOW);
delayMicroseconds(1000);
}
delay(1000); // One second delay
}
Code description
I begin by defining the X, Y and Z step and direction pins with their corresponding Arduino pin connections as specified in the pinout of the CNC shield. I also define the enable pin which is connected to Arduino digital pin 8.
In the setup() section, declare all the motor control pins as digital OUTPUT. You determine the direction of rotation of the stepper motors by setting the direction pins either HIGH or LOW.
Depending on how you connect the motors, setting the DIR pin HIGH will make the motor turn clockwise, and setting it LOW will make the motor turn counterclockwise.
Give the Enable pin a LOW signal to activate the motor driver modules.
In the loop section, I have used these four lines of code below to send a pulse to the step pin resulting in one microstep. A pulse is got by making the output HIGH, waiting a bit then turning it LOW and waiting again.
digitalWrite(stepX, HIGH);
delayMicroseconds(1000);
digitalWrite(stepX, LOW);
delayMicroseconds(1000);
The for loop repeats the above lines of code 800 times, which is 800 steps. The stepper motors I am using make 200 steps per revolution meaning that this will give four revolutions if the motor driver is set to full step resolution.
The number of revolutions made by each motor depends on the microstep resolution of the corresponding motor driver. For example, in ½ step resolution, the motor will make two revolutions.
Determining the speed of rotation.
You determine the speed of rotation of the stepper motors by setting the frequency of the pulses sent to the step pins, which you control using the delayMicroseconds() function. The shorter the delay, the higher the frequency and therefore the faster the motor runs.
When you upload this code and power the CNC shield, the X, Y, and Z-axis motors will begin rotating one at a time, making a given number of revolutions based on the microstep resolution set for the motor driver.
What is GRBL?
GRBL is an open-source software library written in G-code that controls machine motion, such as in CNC machines. This library enables us to be able to use an Arduino UNO to operate CNC machines and any other machine that has 3-axes.
How to install GRBL on Arduino
First, you need to download GRBL .ZIP file from Github
After downloading, open the gbrl-master.zip file and extract the files. Then go to the extracted folder, and within the grbl-master folder, copy the “grbl” folder and paste it in your Arduino Libraries folder which is normally located in Documents > Arduino > Libraries
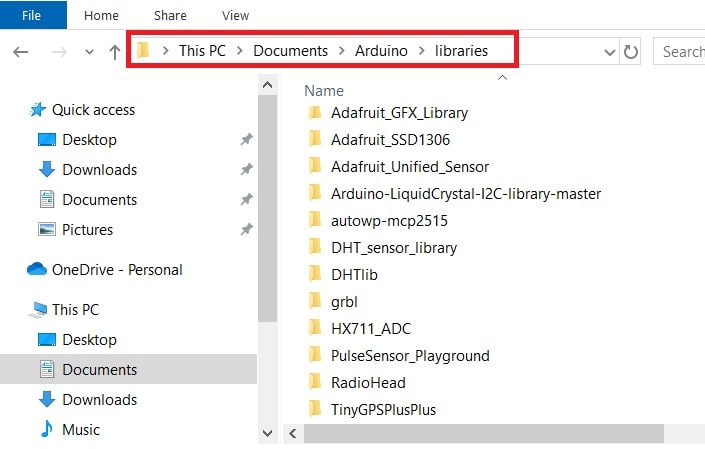
Disabling Z-axis
If you are going to use a machine with only the X and Y axes then you need to disable the Z-axis.
In this case you will have to find this section of code within the “config.h” file:

Then edit the above lines of code to:

You can see that the step for homing the Z-axis has been removed, and now you only home the X-axis and the Y-axis.
Next, in the Arduino IDE go to File > Examples > grbl > grblUpload.
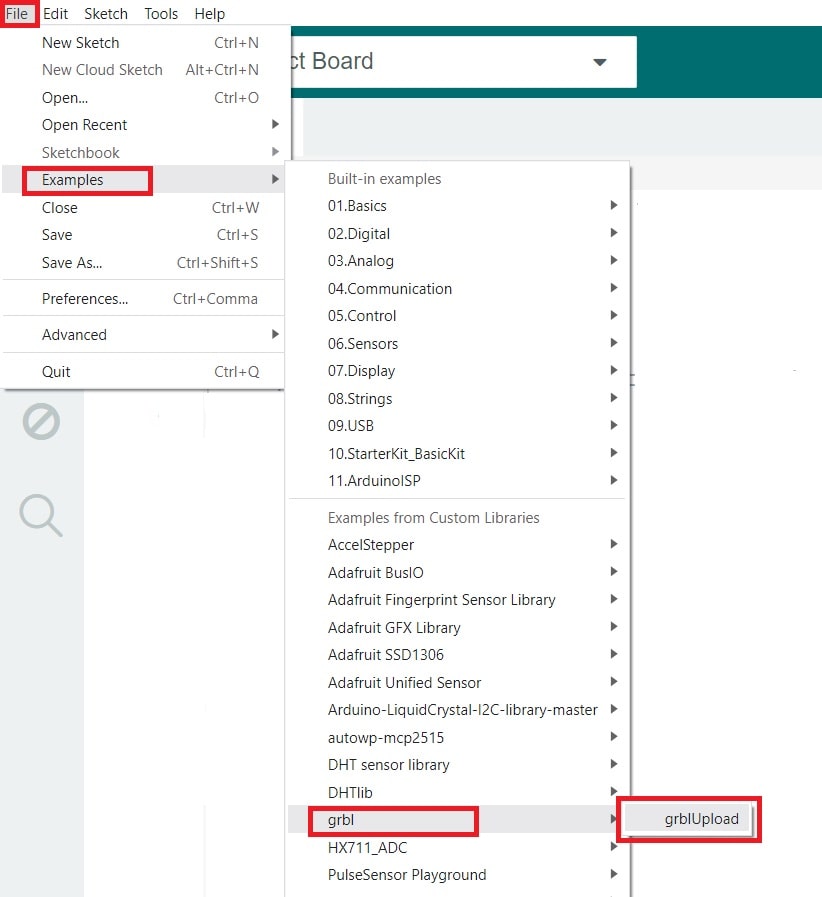
A new code sketch will open which at first sight may look weird because its only one line of code!
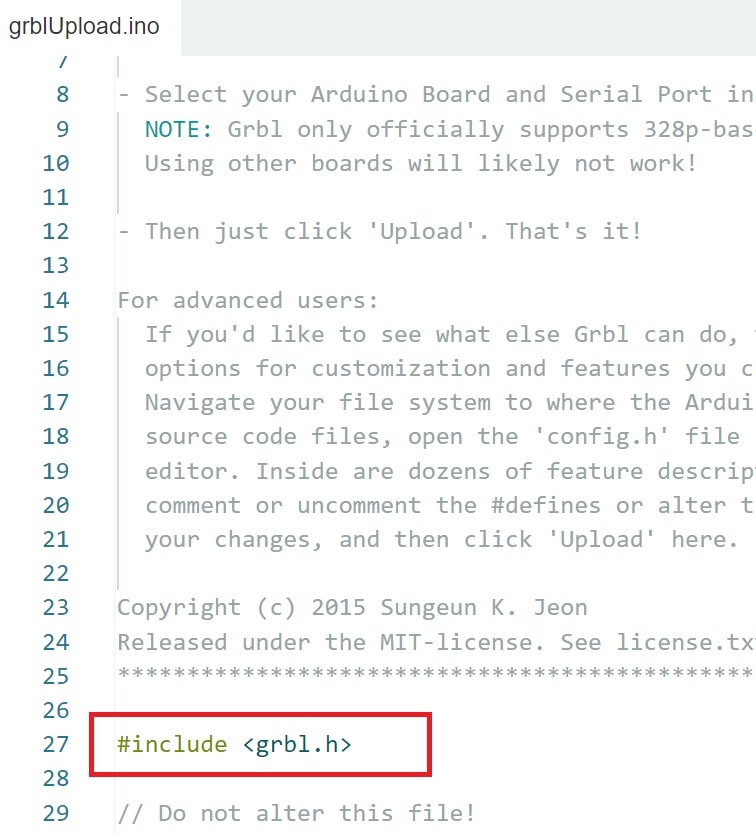
Just select the Arduino board and COM port you are using and upload this code to install the GRBL firmware to the Arduino board.
GRBL Controller
After installing GRBL software, Arduino is now able to read G-code and can control a CNC machine using this code. However, we also need a user interface to be able to send G-code commands to Arduino.
There are a number of open source and commercial G-code controller software but I will use the Universal G-code Sender. (UGS).
You will need to first download the Universal G-code Sender as a zip folder and extract the files. Open the extracted folder and look for the bin folder where you will find the executable file to open UGS.
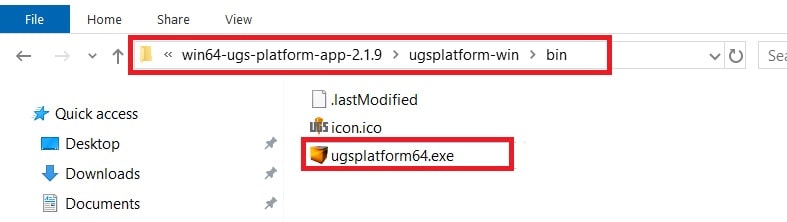
Once you install and open the Universal G-Code Sender, connect your CNC shield to your computer via USB. Then, check the top menu to set the firmware to GRBL, the baud rate to 115200, and select the correct COM port for the shield. Then click on “Connect”.
Do not turn on the 12 -36V CNC Shield power supply just yet!
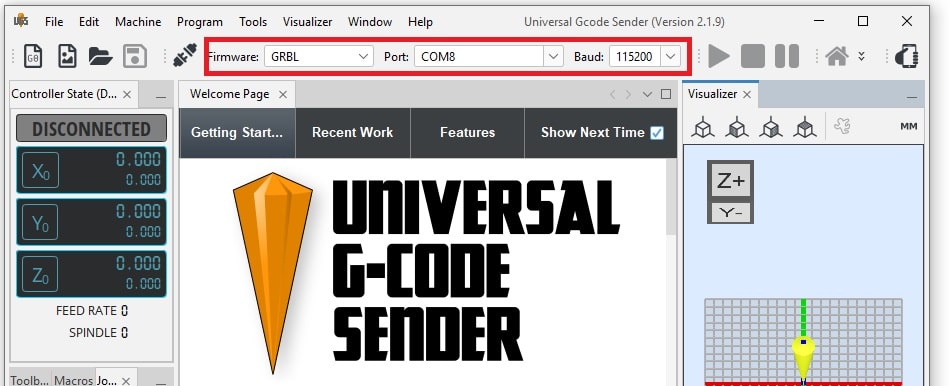
The Connect button is next to the Firmware label and looks like a plug going into an extension cord. If everything is well setup and you press the Connect button, it should get “plugged in” and turn orange.
Now you can power up your CNC shield and begin controlling the stepper motors using the Universal G-code Sender.
You can watch the video below where I have demonstrated the basic use of GRBL and the Universal G-code sender to control stepper motors using the Arduino CNC Shield.