MLX90614 Non-Contact Infrared Temperature Sensor with Arduino.
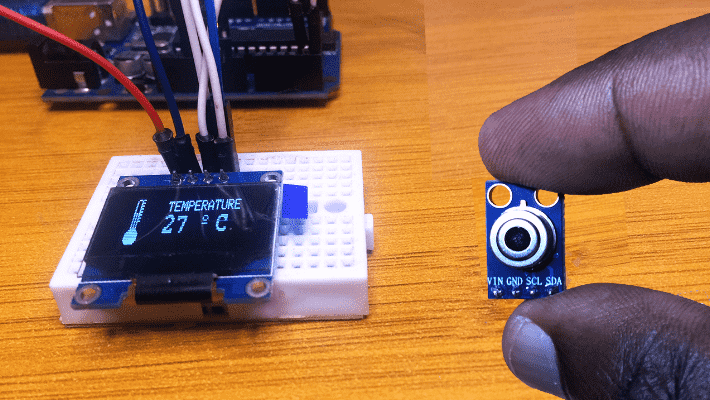
In previous tutorials I have looked at a number of temperature sensors like LM35, DS18B20, DHT11, BME280 and others. These sensors are classified as contact sensors because they measure temperature by making contact with the object whose temperature is to be measured.
Non-contact temperature sensors like the MLX90614 are used in situations where you need to measure temperature of surfaces without necessarily physically touching them. For example, when measuring body temperature using temperature guns, in infrared thermometers for taking temperature of air conditioning units and engine cooling systems.
How does the MLX90614 non-contact Infrared Temperature sensor work?
The MLX90614 sensor measures temperature using infrared radiation emitted by bodies.
The sensor is made up of an infrared thermopile detector and a Digital Signal Processing unit with low noise amplifier and a high resolution 17-bit Analog to Digital Converter. These components are inside a TO-39 metallic casing.
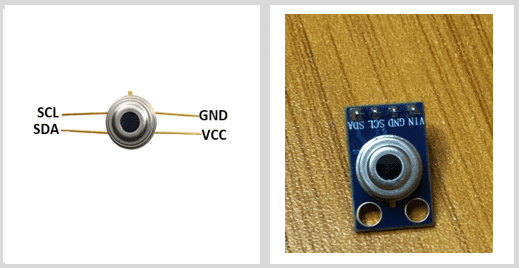
This sensor measures temperatures in the range -40⁰C to 125⁰C for ambient temperature and -70⁰C to 380⁰C for object temperature. The ambient temperature is the temperature of the air around the sensor itself. Object temperature is the average temperature of the surface the sensor is pointed at.
The MLX90614 offers a standard accuracy of ±0.5˚C around room temperatures. There is also a special version of this sensor for medical applications with an accuracy of ±0.2˚C in a limited temperature range around the human body temperature.
The MLX90614 contactless temperature sensor has a 90⁰ field of view therefore the measured value is the average temperature of all the objects in the field of view of the sensor.
In case you need more detailed explanation of the properties of this sensor you can have a look at the datasheet.
Connecting the MLX90614 non-contact temperature sensor to Arduino.
This sensor uses I2C communication and is therefore connected to microcontrollers like Arduino through the I2C pins. Different Arduino boards have different I2C pins but since I am using Arduino UNO, the I2C pins are A4 and A5 for Data (SDA) and Clock (SCL) pins respectively.
The MLX90614 uses 0x5A as the fixed 7-bit I2C address therefore you can only connect one sensor per microcontroller.
If the sensor is not on a breakout board, then you need to include 10kΩ pull-up resistors across the I2C data lines and a 0.1µF capacitor across the power line. For the breakout board the connection is straight forward since these components have already been included on the board.
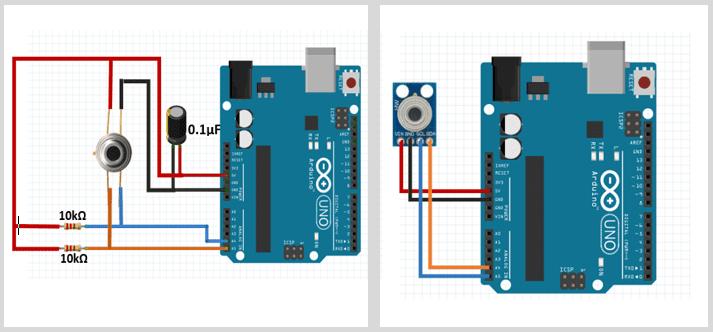
Power supply considerations.
The MLX90614 comes in two variants, that is, the MLX90614Axx version which uses power supply of 4.5V to 5V and the MLX90614Bxx version that uses 2.6V to 3.6V. Therefore, when connecting the sensor to Arduino you need to check the version in order to use the correct power supply.
The breakout board has a 662K 3V regulator IC which accepts input voltages of either 3.3V or 5V. If the input is 3.3V, the output is 3V due to low drop out voltage of this IC and when the input is 5V the output is 3.3V.
Including the MLX90614 Library in Arduino IDE.
To be able to read this sensor’s data using Arduino, you need to first install the Adafruit_MLX90614.h library. This library can be downloaded from GitHub.
After downloading the zip folder from GitHub, you need to unzip this folder and rename the uncompressed folder from “Adafruit-MLX90614-Library” to “Adafruit_MLX90614”.
Then place the Adafruit_MLX90614 folder in the director path for other libraries used by your Arduino IDE and restart the Arduino IDE. For example, in my case this folder will be placed in Documents/Arduino/Libraries
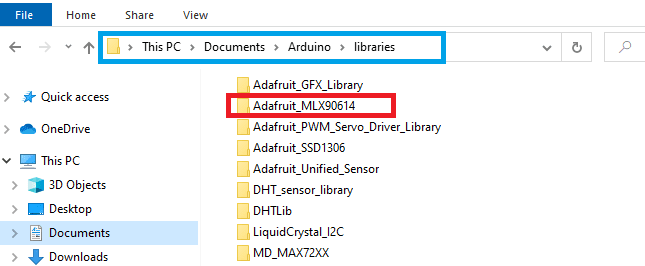
Testing the sensor using the “mlxtest” example.
After installing the Adafruit_MLX90614 library, go to; File->Examples->Adafruit_MLX90614->mlxtest
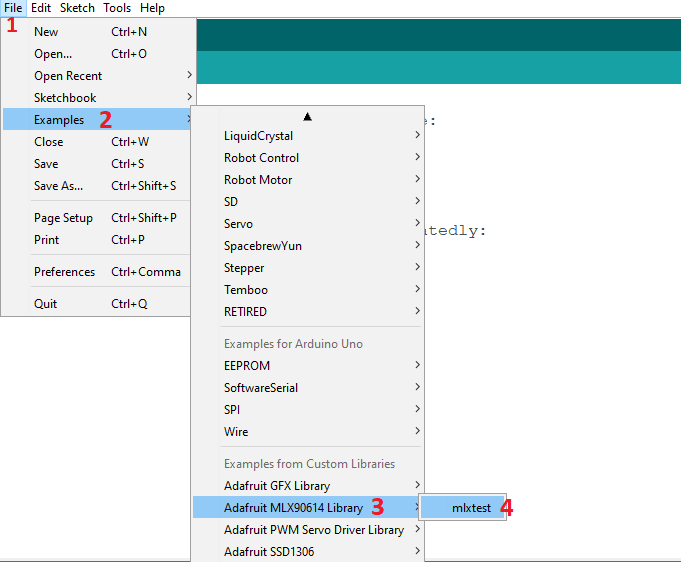
When this code is uploaded to the Arduino, you should be able to see the readings for the ambient and object temperature on the Serial monitor.
Ambient temperature is the temperature of the air around the sensor. The object temp is what its measuring in the 90-degree field of view.
readAmbientTempC()
and readAmbientTemp()
functions are for getting the ambient temperature in degrees Celsius and Fahrenheit respectively.
readObjectTempC()
and readObjectTempF()
functions get the object temperature in degrees Celsius and Fahrenheit respectively.
Displaying MLX90614 Temperature sensor readings on I2C OLED.
We can be able to show the readings of the MLX90614 non-contact temperature sensor on a number of displays. Below I will demonstrate how this sensor can be connected to the 128×32 I2C OLED.
Before proceeding, you can make reference to another post about how to use the I2C OLED with Arduino;
The connection is as shown in the schematic. Both the sensor and OLED use I2C communication and will therefore have their Data and Clock pins attached to pins A4 and A5 of Arduino respectively and also share the ground and power lines.
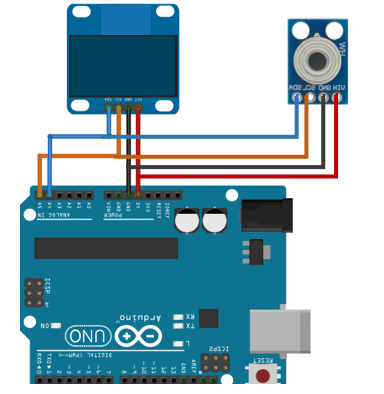
Code for MLX90614 sensor with I2C OLED.
#include <Wire.h>
#include <Adafruit_GFX.h> // Include core graphics library for the display
#include <Adafruit_SSD1306.h> // Include Adafruit_SSD1306 library to drive the display
#include <Fonts/FreeMonoBold9pt7b.h> // Add a custom font
#include <Adafruit_MLX90614.h> //for infrared thermometer
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 32 // OLED display height, in pixels
#define OLED_RESET -1
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
Adafruit_MLX90614 mlx = Adafruit_MLX90614(); //for infrared thermometer
int temp; // Create a variable to have something dynamic to show on the display
void setup()
{
delay(100); // This delay is needed to let the display to initialize
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // Initialize display with the I2C address of 0x3C
display.clearDisplay(); // Clear the buffer
display.setTextColor(WHITE); // Set color of the text
mlx.begin(); //start infrared thermometer
}
void loop()
{
temp++; // Increase value for testing
if(temp > 43) // If temp is greater than 150
{
temp = 0; // Set temp to 0
}
temp = mlx.readObjectTempC(); //comment this line if you want to test
display.clearDisplay(); // Clear the display so we can refresh
// Print text:
display.setFont();
display.setCursor(45,5); // (x,y)
display.println("TEMPERATURE"); // Text or value to print
// Print temperature
char string[10]; // Create a character array of 10 characters
// Convert float to a string:
dtostrf(temp, 4, 0, string); // (variable, no. of digits we are going to use, no. of decimal digits, string name)
display.setFont(&FreeMonoBold9pt7b); // Set a custom font
display.setCursor(22,25); // (x,y)
display.println(string); // Text or value to print
display.setCursor(90,25); // (x,y)
display.println("C"); // Text or value to print
display.setFont();
display.setCursor(78,15); // (x,y)
display.cp437(true);
display.write(167);
// Draw a filled circle:
display.fillCircle(18, 27, 5, WHITE); // Draw filled circle (x,y,radius,color). X and Y are the coordinates for the center point
// Draw rounded rectangle:
display.drawRoundRect(16, 3, 5, 24, 2, WHITE); // Draw rounded rectangle (x,y,width,height,radius,color)
// It draws from the location to down-right
// Draw ruler step
for (int i = 3; i<=18; i=i+2){
display.drawLine(21, i, 22, i, WHITE); // Draw line (x0,y0,x1,y1,color)
}
//Draw temperature
temp = temp*0.43; //ratio for show
display.drawLine(18, 23, 18, 23-temp, WHITE); // Draw line (x0,y0,x1,y1,color)
display.display(); // Print everything we set previously
}
Most of the code above is for displaying the sensor readings on OLED. If you need further reference on how the various functions for displaying information on the OLED work, please make reference to the post below where I go into detail on how to use the I2C OLED with Arduino.
The dtostrf() function converts a float variable to a char array or ASCII representation and store it as a string so that it can easily be printed.
This function takes four input parameters.
dtostrf(floatVar, StringLengthIncDecimalPoint, numVarsAfterDecimal, charBuf)
- floatVar - float variable which we want to convert.
- StringLengthIncDecimalPoint - This is the total length of the string that will be created including the decimal places
- numVarsAfterDecimal - the number of digits to be printed after the decimal point.
- charBuf - the array to store the results
Applications of the MLX90614 Non-contact Temperature sensor.
This sensor and it’s variants have a number of applications for example;
- Used in temperature guns for measuring body temperature
- Industrial temperature control of moving parts like in engine cooling systems
- Used as temperature sensing element for residential, commercial and industrial building air conditioning In automotive blind angle detection and wind shield defogging
- Temperature control in printers and copiers
- Thermal relays and alerts for fire detection systems
- Used for movement detection in security systems
- Multiple zone temperature control in smart homes