Force Sensing Resistor (FSR) with Arduino.
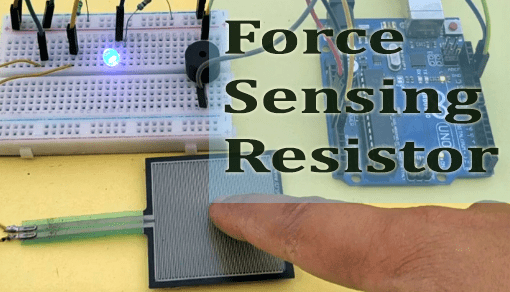
Force Sensing Resistors act as pressure sensors used in a number of devices like electronic drums, mobile phones, handheld gaming devices and many other applications. Although these sensors are easy to use and great for sensing pressure, they are not very accurate and cannot be used in weighing scales. In search applications we would consider using a load cell.
Hardware overview of FSR.
Force sensing resistors come in various shapes and sizes depending on the amount of force the device can withstand. The most common types of FSR are FSR 402 and 406 from Interlink.
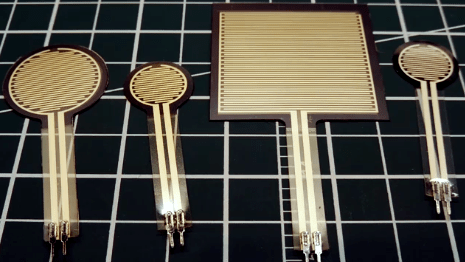
Force sensing resistors consist of a semi-conductive material between two thin substrates. There are two types of force sensing resistor, Shunt Mode and Thru Mode.
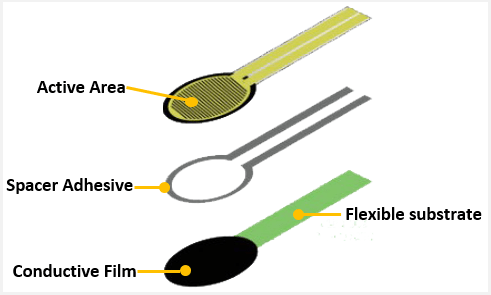
Shunt mode force sensing resistors are polymer thick-film devices consisting of two membranes separated by a thin air gap. One membrane has two sets of interdigitated traces that are electronically isolated from one another, while the other membrane is coated with a special textured, resistive ink.
Thru mode force sensing resistors are flexible printed circuits that utilize a polyester film as its two outer substrates. Silver circles with traces are positioned above and below a pressure-sensitive layer, followed by a conductive polymer. An adhesive layer is used to laminate the two layers of the substrate together.
How do Force Sensing Resistors work?
Force sensing resistors are a piezoresistive sensors, that is, they are passive elements that function as a variable resistor in an electrical circuit. The resistance of an FSR depends on the pressure being applied to the sensor whereby the more pressure applied, the lower the resistance.
The characteristic curve below shows the Resistance vs Force for the FSR 402 sensor.
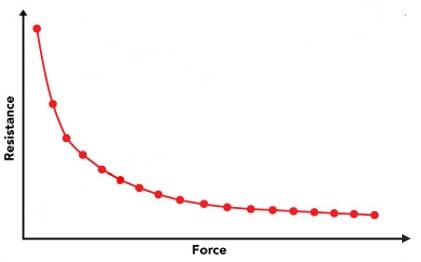
The curve shows that the resistance is inversely proportional to the applied force.
When no force is applied, the sensor is open circuit with a very high resistance. As a little bit of pressure is applied, there is a very large resistance drop and then the resistance gradually becomes inversely proportional to the applied force until a saturation point is reached where an increase in force yields very little to no decrease in resistance.
How to test if the FSR is working properly
To find out whether your FSR is working correctly simply connect it to a multimeter. You can use alligator test leads to connect the exposed leads of the sensor to a multimeter and put the multimeter in resistance (Ω) measuring mode. If the FSR is working properly, you should see the resistance value on the multimeter change when you press the sensor.
Connecting FSR to Arduino.
The force applied to FSR can be measured using a microcontroller like Arduino by creating a voltage divider circuit with the FSR and a pull-down resistor. This circuit creates a variable voltage output that can be read by the analog to digital converter (ADC) of the microcontroller as illustrated below.
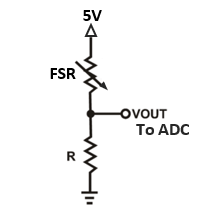
The output voltage Vout is the voltage drop across the pull-down resistor R and not across the FSR therefore the output voltage measured by a microcontroller is given by;
Vout = Vcc x R / (R + Rfsr)
The value of the pull-down resistor R determines the FSR force range for example, for a 100g to 10kg FSR, a resistor of 10kΩ works well if the sensor is to be used over the whole range.
The schematic below shows how to connect the force sensing resistor to Arduino. The FSR, like any other resistor, is non-polarized therefore no need for looking for positive or negative side. Simply connect the leads in any orientation you want.
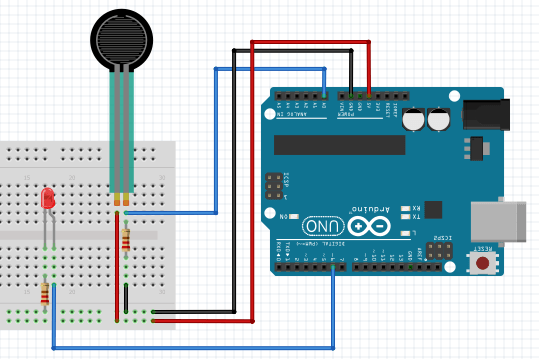
Connect one of the leads of the FSR to Arduino 5V and the other lead to the analog pin A0. The pull-down resistor gets connected between GND and A0.
I have also included an LED with a 150Ω resistor to PWM pin 6 of the Arduino. I will use this LED in some examples later to demonstrate how this sensor can be used to control other electronic components.
FSR Analog Voltage reading
Before applying a force sensing resistor with a microcontroller like Arduino, we need to know it’s out put voltage with reference to the microcontroller’s ADC. In the setup above, the output voltage of the sensor will be between 0 V when no pressure is applied and roughly 5 V when maximum pressure is applied.
Arduino boards have 10-bit analog to digital converter therefore the input voltage of 0 to 5 V will be converted to integer values from 0 to 1023. The code below is uploaded to Arduino to achieve this conversion.
int fsrAnalogPin = 0;
int fsrReading;
void setup(void) {
Serial.begin(9600);
}
void loop(void) {
fsrReading = analogRead(fsrAnalogPin);
Serial.print("Analog reading = ");
Serial.println(fsrReading);
delay(100);
}
When you press the FSR, you will see values between 0 and 1023 on the serial monitor depending on how much force is being applied on the sensor.
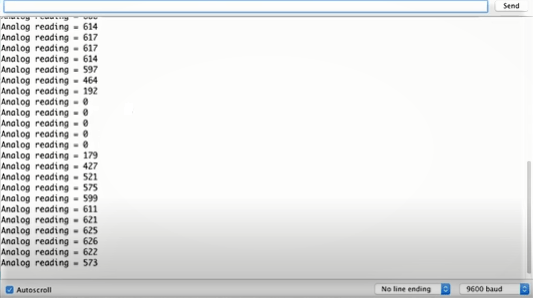
Using FSR as a switch with Arduino.
After mapping the FSR voltage output to the Arduino ADC readings, you can now use this sensor in a number of applications. The first example will be using the FSR sensor as a toggle switch to switch an LED on and off.
Code for using FSR as a toggle switch with Arduino.
#define fsrpin A0
#define ledpin 6
int fsrreading; // The current reading from the FSR
int state = HIGH; // The current state of the output pin
int previous = 0; // The previous reading from the FSR
long time = 0; // The last time the output pin was toggled
long debounce = 40; // The debounce time, increase if the output flickers
void setup() {
Serial.begin(9600);
pinMode(ledpin, OUTPUT);
}
void loop() {
fsrreading = analogRead(fsrpin);
Serial.println(fsrreading);
if (fsrreading > 600 && previous < 600 && millis() - time > debounce) {
if (state == HIGH)
state = LOW;
else
state = HIGH;
time = millis();
}
digitalWrite(ledpin, state);
previous = fsrreading;
}
When the above code sketch is uploaded to Arduino, the LED will toggle on and off whenever you press the FSR. The state of the LED changes whenever the analog input pin value exceeds 600.
Controlling LED brightness with FSR and Arduino.
LED brightness can also be controlled using the FSR by varying the voltage at the PWM pin where the LED is connected depending on how much the sensor is pressed. This is achieved using the code below.
int fsrAnalogPin = 0; // FSR is connected to analog 0
int LEDpin = 6; //LED on pin 6 (PWM pin)
int fsrReading; // analog reading from the FSR resistor divider
int LEDbrightness;
void setup(void) {
Serial.begin(9600); // We'll send debugging information via the Serial monitor
pinMode(LEDpin, OUTPUT);
}
void loop(void) {
fsrReading = analogRead(fsrAnalogPin);
Serial.print("Analog reading = ");
Serial.println(fsrReading);
LEDbrightness = map(fsrReading, 0, 1023, 0, 255);
analogWrite(LEDpin, LEDbrightness);
delay(100);
}
The map function is used to change the range 0 -1023 used by the Arduino ADC to the range 0-255 which is used at the PWM pin where the LED is connected.
With this code, the harder you press the FSR, the brighter the LED gets.