How MQ2 Gas Sensor works with Arduino.
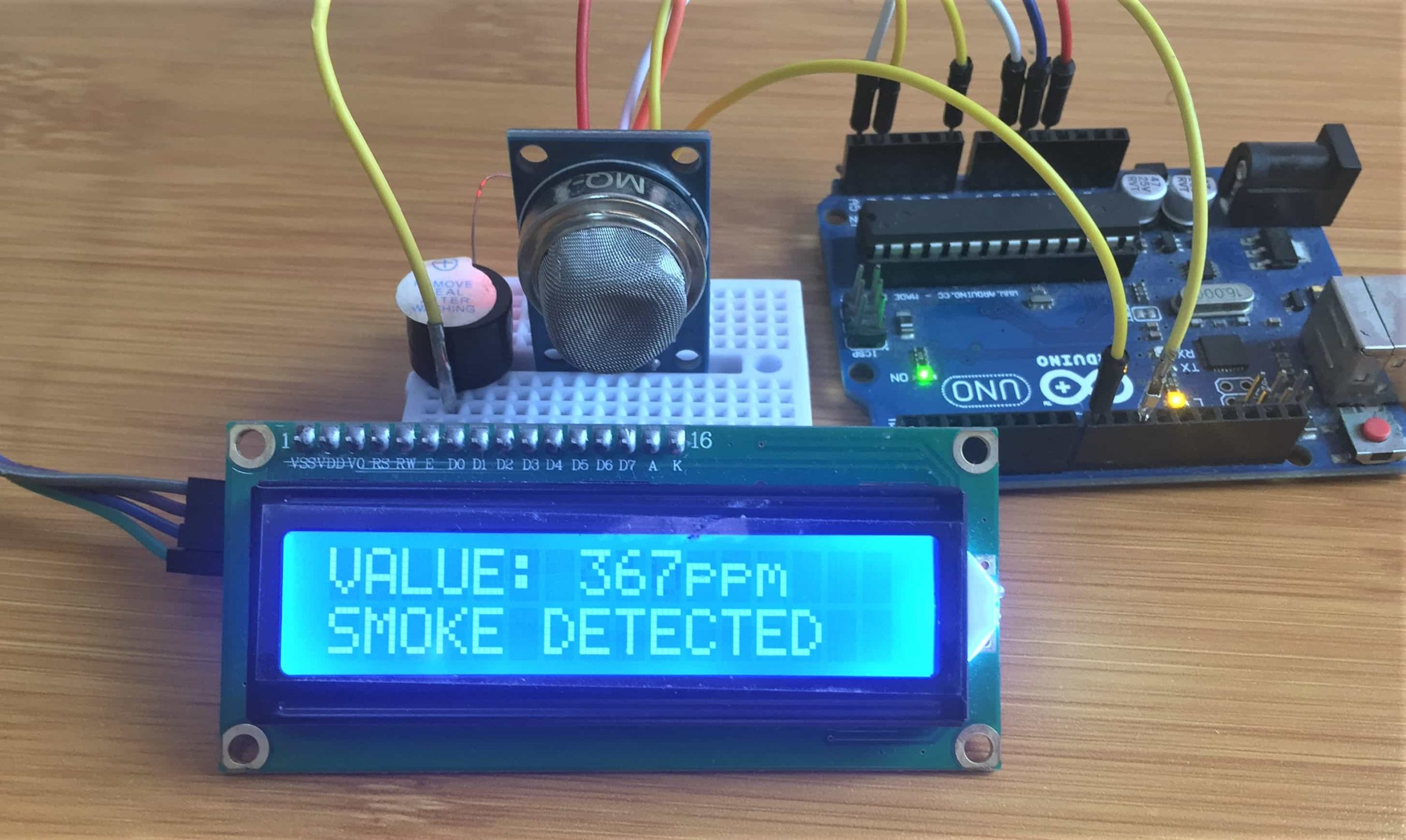
The MQ2 gas sensor is used for detecting the levels of various gases around the area, so you can utilize this sensor as a gas leak monitoring system, in fire alarms and air quality monitoring for homes, businesses or factories.
MQ2 gas sensor description.
The MQ2 gas sensor is a chemiresistor. That is, it contains a sensing material whose resistance changes when it comes in contact with a gas. This change in the value of resistance is used for the detection of gas.
The sensing element is made of mainly aluminium-oxide based ceramic, coated with Tin dioxide, enclosed in a stainless steel mesh. This tutorial is mainly for showing the physical features of this sensor and how to connect it to the Arduino.
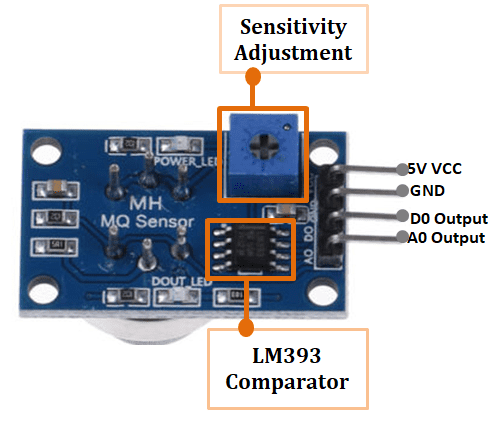
VCC : Module power supply. Connect it to 5V output of Arduino.
GND : Ground Pin. Connect to GND pin of the Arduino.
DO : Digital Output representation of the gas.
A0 : Analog Output voltage proportional to the concentration of gas.
The LM393 comparator is for checking if the analog pin (A0) has reached the threshold value set by sensitivity adjustment potentiometer. When it goes past the threshold, the digital pin (D0) will go HIGH.
The major downside of this sensor is that it only senses the concentration of gases in the air such as LPG, propane, methane, hydrogen, alcohol, smoke and carbon monoxide. However it can not distinguish among the different types of gases. For that you may need other types of gas sensors like MQ-6, M-306A and AQ-3 which detect specific type of gases.
Making a smoke detection system using MQ2 gas sensor with Arduino.
The mq2 gas sensor is connected to the Arduino board as shown in the schematic below. Pins AO and DO of the sensor are connected to Arduino pins A0 and 8 respectively.
We have included a buzzer on pin 10 for sounding an alarm when the threshold has been breached and an I2C LCD is also included to display the value of concentration of the smoke in ppm.
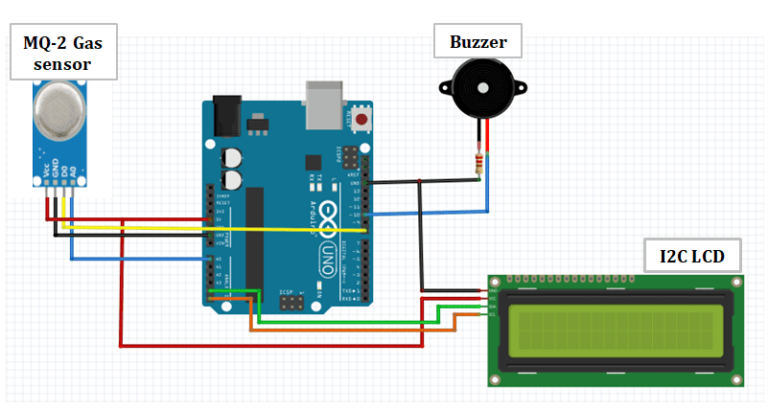
Code for smoke detection system using MQ2 Gas sensor.
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 2, 1, 0, 4, 5, 6, 7, 3, POSITIVE);
#define MQ2pin (0)
int buzzer = 10;
int sensorValue; //variable to store sensor value
void setup()
{
pinMode(buzzer, OUTPUT);
lcd.begin(16,2);
lcd.backlight();
lcd.setCursor(0,0);
lcd.print(" GAS SENSOR ");
delay(1000);
lcd.setCursor(0,1);
lcd.print(" WARMING UP ! ");
delay(20000); // allow the MQ-6 to warm up
}
void loop()
{
lcd.clear();
sensorValue = analogRead(MQ2pin); // read analog input pin 0
lcd.setCursor(0,0);
lcd.print("VALUE: ");
lcd.print(sensorValue);
lcd.print("ppm");
if(sensorValue > 300)
{
lcd.setCursor(0,1);
lcd.print("SMOKE DETECTED");
tone(buzzer, 500, 2000);
}
else
{
lcd.setCursor(0,1);
lcd.print(" NO SMOKE");
noTone(buzzer);
}
delay(2000); // wait 2s for next reading
}
When this code is uploaded to the Arduino board, the sensor will have to first stay dormant for about 20 minutes during which time the sensing material is being warmed up.
After the warm up we can be able to see the concentration of the smoke on the LCD and if the concentration goes above the set threshold, in this case 300 then the alarm will sound.